ASP.NET MVC Actions
ASP.NET MVC Actions executes requests received at Controllers and generates corresponding responses and results for the requests. Default response of Actions are ActionResult.
Example of ASP.NET MVC Actions :
We created a Controller named HomeController in our previous tutorial, continuing on that let’s try to understand the ASP.NET MVC Actions.
Our HomeController :
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace MyMvcApplication.Controllers { public class HomeController : Controller { // // GET: /Home/ public String Index() { return "Hello, I'm a simple ASP.NET MVC CONTROLLER."; } } }
If you run this, you can type localhost/Home/Index or localhost/Home/ in url section of your browser and this action will be called.
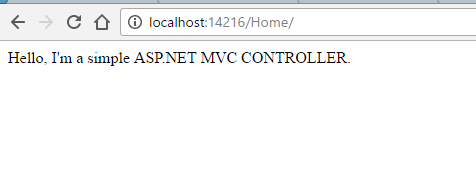
ASP.NET MVC Actions overview – Running the website
Index method will be default ActionResult if no action method is called.
Now, let’s add another Action to our controller
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace MyMvcApplication.Controllers { public class HomeController : Controller { // // GET: /Home/ public String Index() { return "Hello, I'm a simple ASP.NET MVC CONTROLLER."; } public String Date() { return System.DateTime.Now.ToString(); } public String DaysOfWeek() { return "<ol><li>Sunday</li><li>Monday</li>"+ "<li>Tuesday</li><li>Wednesday</li>" + "<li>Thrusday</li><li>Friday</li>" + "<li>Saturday</li>" + "</ol>"; } } }
On calling /home/Date/ you will be getting current date and time as output and on calling /home/DaysOfWeek you will get list of days supplied above.
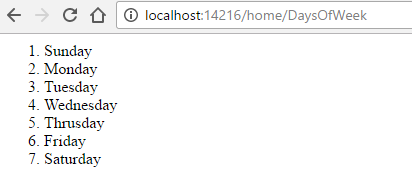
ASP.NET MVC Actions – Example 2
Now we will create a Action method which will show addition of two numbers. From App_start folder open the RouteConfig.cs file and add a new route to it as below :
RouteConfig.cs:
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; using System.Web.Routing; namespace MyMvcApplication { public class RouteConfig { public static void RegisterRoutes(RouteCollection routes) { routes.IgnoreRoute("{resource}.axd/{*pathInfo}"); routes.MapRoute( name: "Default", url: "{controller}/{action}/{id}", defaults: new { controller = "Home", action = "Index", id = UrlParameter.Optional } ); routes.MapRoute( name: "Home", url: "{controller}/{action}/{a}/{b}", defaults: new { controller = "Home", action = "Calculate", a = UrlParameter.Optional, b = UrlParameter.Optional } ); } } }
And in our HomeController.cs add another Action method as below :
HomeController.cs:
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; namespace MyMvcApplication.Controllers { public class HomeController : Controller { // // GET: /Home/ public String Index() { return "Hello, I'm a simple ASP.NET MVC CONTROLLER."; } public String Date() { return System.DateTime.Now.ToString(); } public String Calculate(String a, String b) { return Convert.ToString(Convert.ToInt32(a) + Convert.ToInt32(b)); } public String DaysOfWeek() { return "<ol><li>Sunday</li><li>Monday</li>" + "<li>Tuesday</li><li>Wednesday</li>" + "<li>Thrusday</li><li>Friday</li>" + "<li>Saturday</li>" + "</ol>"; } } }
Now when we call Calculate method along with two supplied parameters we will get result of both the parameters values:
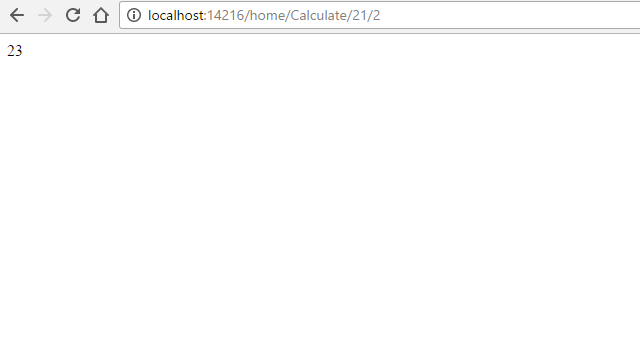
ASP.NET MVC Actions – Example 2