In this post we will see how we can use JQuery Datepicker on ASP.NET MVC web forms. We will create a simple form with few MVC textboxes and apply jquery datepicker on one of it. After user selects the Date from the picker we will parse date and convert it into different format and show in on MVC view. So let’s see.
DOWNLOAD SOURCE CODE.
In your MVC project create a new Model class with name Students.cs and edit it as below:
YourProject > Models > Students.cs:
namespace MVCWeb25.Models { public class Students { public string studentName { get; set; } public string studentClass { get; set; } public string studentDOB { get; set; } } }
Now in your HomeController.cs add Index method (along with HttpPost method) as below:
Controllers > HomeController.cs:
using MVCWeb25.Models; using System; using System.Web.Mvc; namespace MVCWeb25.Controllers { public class HomeController : Controller { public ActionResult Index() { return View(); } [HttpPost] public ActionResult Index(Students students) { String date = DateTime.ParseExact(students.studentDOB, "dd/M/yyyy", System.Globalization.CultureInfo.InvariantCulture).ToString("dd-MMM-yyyy"); ViewBag.Message = date; return View(students); } } }
Add a View for Index method and edit it as below:
Views > Home > Index.cshtml:
@model MVCWeb25.Models.Students @{ ViewBag.Title = "Home Page"; Layout = null; } <link href="~/Content/bootstrap.css" rel="stylesheet" /> <link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-1.12.4.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> <style> input[type="text"] { padding: 3px; border-radius: 5px; border: 1px solid #929292; } </style> @using (Html.BeginForm()) { <div style="padding:5px;"> <h2>JQuery Datepicker Sample on MVC Web Forms</h2> <hr /> <h3> Student Details: </h3> <table class="table-condensed"> <tr> <td> Name: </td> <td> @Html.TextBoxFor(m => m.studentName) </td> </tr> <tr> <td> Class: </td> <td> @Html.TextBoxFor(m => m.studentClass) </td> </tr> <tr> <td> DOB: </td> <td> @Html.TextBoxFor(m => m.studentDOB, new { @id = "dob" }) </td> </tr> <tr> <td colspan="2" align="left"> <input type="submit" value="submit" name="Add" class="btn btn-success" /> </td> </tr> </table> <hr /> @ViewBag.Message <script type="text/javascript"> $(document).ready(function () { $("#dob").datepicker({ dateFormat: "dd/mm/yy", changeMonth: true, changeYear: true, yearRange: "-20:+0" }); }); </script> </div> }
The javascript will add the JQuery picker to our “dob” textbox (we have given the ID to the Student DOB textbox). When we focus on the DOB Html.TextBoxFor textbox we will see the JQuery datepicker to select the date.
Output:
DOWNLOAD SOURCE CODE.
Also see :
Also see:
Custom CSS design for JQuery Datepicker
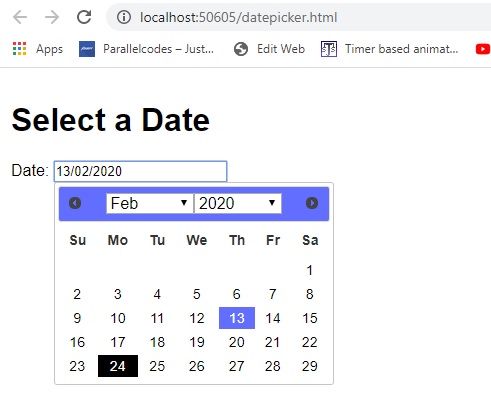
JQuery Datepicker custom design CSS